8.5.2 Insert New Records to the Faculty Table Using JavaServer Pages and Java Beans
To use JavaServer Pages and Java bean techniques to insert a new record into the Faculty Table, we need to perform the following operations:
1) Create a new Java bean class file, FacultyInsertBean.java, to handle the new fac-ulty record insertion actions.
2) Modify the model controller page, FacultyProcess.jsp, to handle faculty data collec-tion and assist with data insertion operations.
First let’s create a new Java bean class file, FacultyInsertBean.java, to handle the new faculty record insertion actions.
8.5.2.1 Create a New Java Help Class File, FacultyInsertBean.java
To create our Java bean class file, FacultyInsertBean.java, to handle faculty record insertion, right-click on our project, JavaWebOracleInsert, in the Projects win-dow and select the New > Java Class item from the popup menu to open the New Java Class wizard. Enter FacultyInsertBean in the Class Name field and select the JavaWebOracleSelectPackage from the Package combo box. Click on the Finish but-ton to create the new Java bean class file.
Now open the code window of the new FacultyInsertBean.java class file by clicking on the Source button at the top and enter the code shown in Figure 8.81 into this window.
Let’s have a closer look at this code to see how it works.
A. Some necessary Java libraries are imported first, since we need to use some components located in those libraries.
B. The constructor of this bean class is generated with the database connection operations included, which are identical to the code in the FacultyQuery.java constructor.
C. A new method, InsertFaculty(), is generated with a String array as the argument that contains a new record to be inserted into the Faculty Table in our sample database.
D. A local integer variable, numInsert, is used to hold the returned insertion result. Usually, it is equal to the number of records that have been inserted into the Faculty Table. A FileInputStream object, fis, is also created and works as a handler for converting our faculty image to a FileInputStream before it can be written or inserted into our database. A new File object, fimage, is declared with the name of our faculty image file as the target since the String variable, new Faculty [7], contains the name of a selected faculty image to be inserted into the database.
E. The insert string is created with eight positional parameters represented by question marks in the query string.
F. A try-catch block is used to perform the faculty record insertion action. First a Prepared-Statement object is created with the query string as the argument.
G. Then seven elements in the String array newFaculty[], which are equivalent to seven pieces of new faculty information, are assigned to seven positional parameters. The point
to be noted is that the order of those seven elements must be identical to the order of col-umns represented in the query string and in the Faculty Table in our sample database.
H. Another try-catch block is used to create a new handler for the converted FileInputStream object with our faculty image as the input.
I. The faculty image is inserted by calling the setBinaryStream() method with the handler of our FileInputStream as the source.
J. The executeUpdate() method is executed to perform the new record insertion action. The run result, which equals the number of records that have been successfully inserted into the Faculty Table, is returned and assigned to the local integer variable numInsert.
K. The catch block is used to track and collect any possible exceptions during the data insertion action.
L. Finally, the run result is returned to the calling method on the FacultyProcess.jsp page.
Next let’s modify the model controller page FacultyProcess.jsp to handle the faculty data collection and insertion operations.
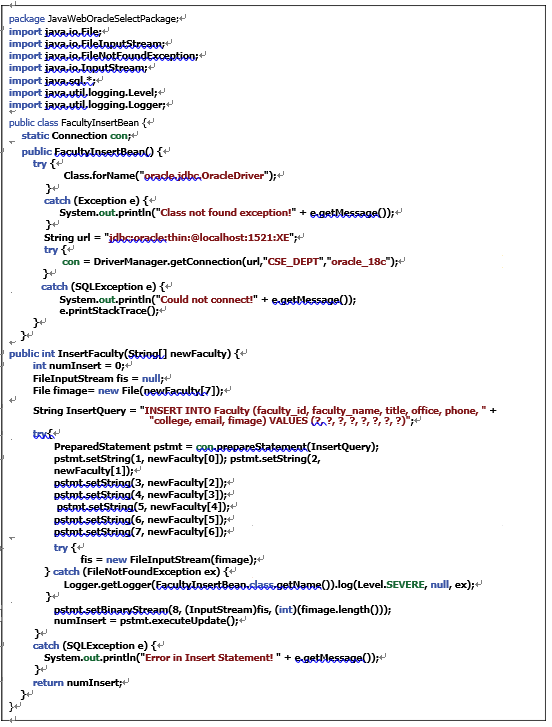
FIGURE 8.81 The code for the new created bean, FacultyInsertBean.java.